UserControls are relatively practical due to their multiple usability. If you create more complex user controls in C#/XAML, e.g. with Visual Studio, and call further dialogs/Windows from the UserControl, then these dialogs or Windows appear somewhere on the screen.
WindowStartupLocation CenterOwner does not work
In order for a dialog to appear within the program interface as much as possible, you can use the following code, which centers the called dialog over the element from which it was called. It is important that the Owner property is set before the call.
this.WindowStartupLocation = WindowStartupLocation.CenterOwner;
The only problem is that WindowStartupLocation does not work in the context of a user control.
dialog.Owner = MyUserControl;
This throws an error:
Cannot implicitly convert type ‘UserControlType’ to ‘System.Windows.Window’
The reason is that the UserControl itself cannot be the Owner, because due to its multiple use it is only one element within a window/dialog. This means that you would have to determine the Parent window of the user control.
UserControl.Parent as Window
Since a UserControl can be an element of another UserControl and this can also be an element of another UserControl, you would have to try to determine the parent via a loop, otherwise this procedure also fails.
Align dialog/window to position of a UserControl
To align a dialog/window, which is called by a user control, with the user control, I use the following code to determine the location on the screen. This is then passed to the dialog/window.
MyDialog dialog = new (MyParameter);
Point p = MyUserControl.PointToScreen(new Point(0, 0));
dialog.Top = p.Y;
dialog.Left = p.X;
dialog.ShowDialog();
“MyUserControl” can also be replaced by a specific element on the UserControl, e.g. a button. Then the dialog will be aligned exactly to this button.
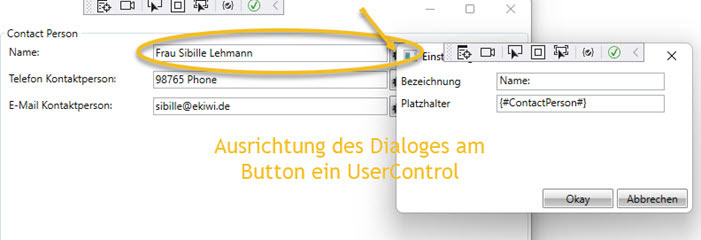